Here are a few short descriptions for a blog on Python objects, depending on the target audience and the specific focus
- “Unlocking Python’s Power: Dive into the World of Objects” – Starts with an intriguing hook and promises an introduction to essential concepts.
- “From Numbers to Robots: Build Anything with Python Objects” – Uses everyday examples to pique interest and highlights the versatility of objects.
- “Mastering the Magic: Demystifying Python Objects and Classes” – Plays on curiosity and positions the blog as a guide to conquering a seemingly complex topic.
For intermediate learners:
- “Beyond Basics: Level Up Your Python with Advanced Object-Oriented Techniques” – Targets a specific desire for growth and showcases the blog’s focus on advanced concepts.
- “Python Objects Demystified: A Deeper Look at Attributes, Methods, and More” – Emphasizes technical details and appeals to learners seeking a comprehensive understanding.
- “Unleash the Potential: Mastering Inheritance, Polymorphism, and Other Object-Oriented Magic” – Highlights advanced topics and suggests the blog will unlock greater coding power.
For a specific focus:
- “From Strings to Lists: Demystifying Built-in Python Objects” – Tailors the description to the specific content covered in the blog.
- “Build Your Own: Custom Classes and Objects for Python Mastery” – Emphasizes the practical application of object-oriented programming.
- “Debug Like a Pro: Understanding Common Python Object Errors” – Targets a specific pain point and positions the blog as a helpful resource.
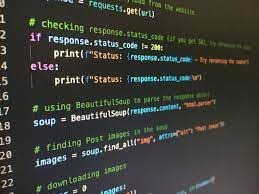
Demystifying Python Objects: A Journey into the Heart of the Language
Welcome, fellow Python enthusiasts, to a journey into the fascinating realm of Python objects! Objects are the building blocks of object-oriented programming (OOP), a powerful paradigm that shapes how we think about and design Python programs.
But what exactly are these mysterious objects? And why should you care about them? Buckle up, because we’re about to dive deep and uncover the secrets that make Python objects tick.
What is an Object?
Imagine an object as a self-contained package. Inside, you’ll find two key ingredients:
- Data: This can be anything from simple numbers and strings to complex structures like lists and dictionaries. Think of it as the object’s internal state, its unique set of characteristics.
- Methods: These are functions specific to the object that can manipulate its data. They act like tools that allow the object to interact with the world and perform specific tasks.
For example, consider a Car
object. Its data might include properties like color, mileage, and fuel level. Methods could be startEngine()
, accelerate()
, and calculateFuelConsumption()
. Each method operates on the car’s data, changing its state or returning information about it.
Creating Objects: Bringing Blueprints to Life
Objects are usually created from classes, which act as blueprints or templates. A class defines the data and methods that all its objects will share. Think of it as a cookie cutter: you can use the same cutter to create many cookies, each with the same basic shape but potentially different details like icing or sprinkles.
Here’s a simple example of a Circle
class and how to create objects from it:
Python
class Circle:
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14 * self.radius**2
circle1 = Circle(5)
circle2 = Circle(10)
print(circle1.area()) # Output: 78.5
print(circle2.area()) # Output: 314
Use code with caution. Learn morecontent_copy
As you can see, both circle1
and circle2
are instances of the Circle
class, inheriting its data (radius) and methods (area). But they have different values for the radius, making them unique circles.
Object Relationships: A Tangled Web
Objects don’t exist in isolation. They can interact with each other in various ways, building complex relationships:
- Inheritance: Classes can inherit from other classes, acquiring their data and methods. This allows for code reuse and specialization. Imagine a
Sedan
class inheriting from theCar
class, adding specific methods likeopenSunroof()
. - Composition: Objects can contain other objects within them. For example, a
Library
object could contain multipleBook
objects. - Association: Objects can simply reference each other without direct ownership. Think of a
Student
object having a reference to aTeacher
object.
These relationships add another layer of complexity and power to object-oriented programming.
Benefits of Using Objects
So, why should you embrace objects in your Python code? Here are some key advantages:
- Modularization: Objects break down your program into smaller, manageable units, making it easier to understand, maintain, and debug.
- Reusability: Code within objects can be easily reused, reducing redundancy and saving you time.
- Flexibility: Objects allow you to create specialized entities with specific behaviors, catering to diverse needs within your program.
- Abstraction: Objects hide the internal details of how things work, exposing only the essential functionalities to the outside world.
Ready to Object-fy Your Code?
Understanding objects is crucial for mastering Python’s object-oriented features. By embracing this paradigm, you can write cleaner, more efficient, and more maintainable code, taking your Python skills to the next level.
Remember, the journey into the world of objects is full of fascinating discoveries. So, keep exploring, experimenting, and don’t hesitate to ask questions along the way!
Bonus Tip: Check out these online resources for further exploration:
- Python official documentation on classes: https://docs.python.org/3/tutorial/classes.html
- Interactive Python tutorial on objects: https://www.w3schools.com/python/
- Fun and challenging exercises to practice object-oriented programming: https://www.practicepython.org/
Happy coding!